摘要
在图像处理中,翻转是一种常见的操作,它可以改变图像的方向或镜像图像。在本文中,我们将学习如何使用 C# 来进行图像翻转,并介绍常用的属性和方法。
正文
图像翻转的常用属性和方法
在 C# 中,我们可以使用 System.Drawing
命名空间中的 Bitmap
类来加载和处理图像。下面是一些常用的属性和方法:
属性
Bitmap.Width
:获取图像的宽度。
Bitmap.Height
:获取图像的高度。
方法
Bitmap.Clone(Rectangle rect, PixelFormat format)
:创建一个图像的副本,并指定副本的像素格式。
Graphics.DrawImage(Image image, Rectangle destRect, int srcX, int srcY, int srcWidth, int srcHeight, GraphicsUnit srcUnit)
:将图像绘制到指定的矩形区域中。
水平翻转图像
下面是一个示例代码,演示如何水平翻转图像:
public class ImageProcessor
{
public Bitmap FlipHorizontal(Bitmap image)
{
// 创建图像的副本,并指定像素格式
Bitmap flippedImage = image.Clone(new Rectangle(0, 0, image.Width, image.Height), image.PixelFormat);
// 水平翻转图像
flippedImage.RotateFlip(RotateFlipType.RotateNoneFlipX);
return flippedImage;
}
}
// 使用示例
ImageProcessor imageProcessor = new ImageProcessor();
Bitmap image = (Bitmap)Image.FromFile("D:\\BaiduSyncdisk\\11Test\\feGsv0kJ6CEBng3.png");
Bitmap flippedImage = imageProcessor.FlipHorizontal(image);
pictureBox1.Image=flippedImage;
垂直翻转图像
下面是一个示例代码,演示如何垂直翻转图像:
public Bitmap FlipVertical(Bitmap image)
{
// 创建图像的副本,并指定像素格式
Bitmap flippedImage = image.Clone(new Rectangle(0, 0, image.Width, image.Height), image.PixelFormat);
// 垂直翻转图像
flippedImage.RotateFlip(RotateFlipType.RotateNoneFlipY);
return flippedImage;
}
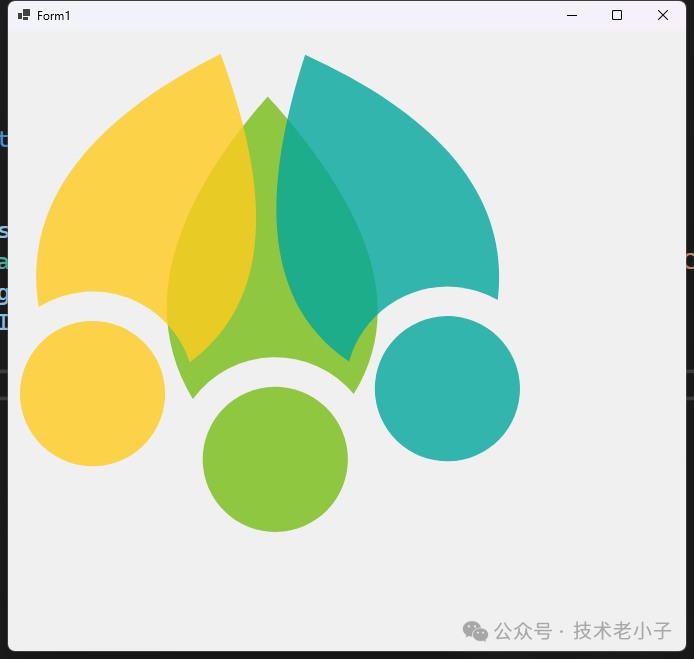
旋转图像
下面是一个示例代码,演示如何旋转图像:
public Bitmap RotateImage(Bitmap image, float angle)
{
// 创建图像的副本,并指定像素格式
Bitmap rotatedImage = image.Clone(new Rectangle(0, 0, image.Width, image.Height), image.PixelFormat);
// 旋转图像
using (Graphics graphics = Graphics.FromImage(rotatedImage))
{
graphics.Clear(Color.White);
graphics.TranslateTransform(image.Width / 2, image.Height / 2);
graphics.RotateTransform(angle);
graphics.TranslateTransform(-image.Width / 2, -image.Height / 2);
graphics.DrawImage(image, new Point(0, 0));
}
return rotatedImage;
}
// 使用示例
Bitmap image = (Bitmap)Image.FromFile("D:\\BaiduSyncdisk\\11Test\\feGsv0kJ6CEBng3.png");
ImageProcessor imageProcessor = new ImageProcessor();
Bitmap rotatedImage = imageProcessor.RotateImage(image, 45);
pictureBox1.Image = rotatedImage;
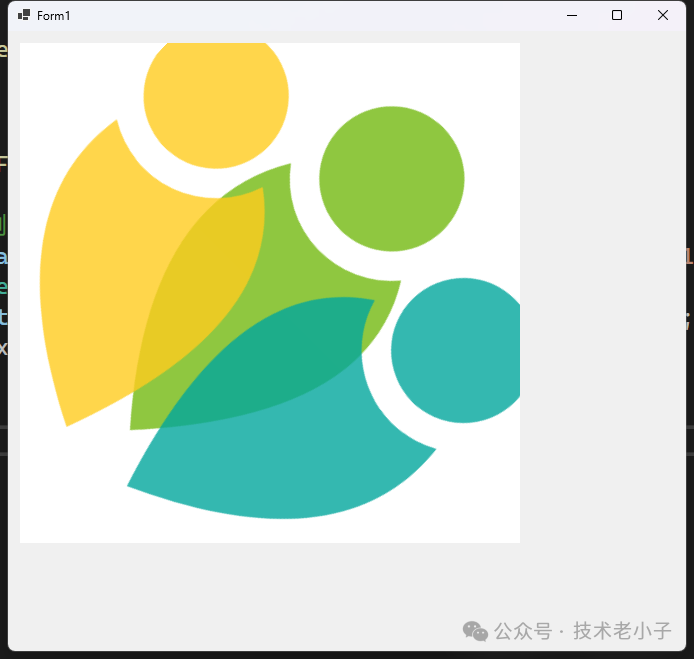
在上面的示例中,我们首先创建了一个图像的副本,并指定像素格式。然后,我们使用 Graphics
类的 TranslateTransform
和 RotateTransform
方法对图像进行旋转。最后,我们返回旋转后的图像副本。
总结
在本文中,我们介绍了如何使用 C# 来进行图像翻转。通过水平翻转、垂直翻转和旋转图像,我们可以实现各种图像处理任务。我们通过示例代码演示了常用的属性和方法,并提供了一些示例来说明其用法。
该文章在 2024/8/29 12:40:51 编辑过